Simple MVC framework in Ruby
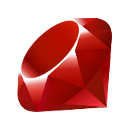
For the beginning we will create a gem by running:
bundle gem simplemvc
then open the file /simplemvc/lib/simplemvc.rb
require "simplemvc/version"
module Simplemvc
# Your code goes here...
class Application
def call(env)
[200, {"Content-Type" => "text/html"}, ["Hello"]]
end
end
end
mkdir blog
cd blog
touch Gemfile
Edit the Gemfile:
source "https://rubygems.org"
gem "simplemvc", path: "../simplemvc"
gem "sqlite3"
To build and install the gem run:
gem build simplemvc.gemspec
gem install simplemvc-0.0.1.gem
bundle
touch config.ru
Edit config.ru
require './config/application.rb'
use Rack::ContentType
app = Blog::Application.new
app.route do
match "/", "home#index"
match "/:controller/:action" # /haha/hehe
end
run app
Create the file /blog/config/application.rb
require 'simplemvc'
$LOAD_PATH << File.join(File.dirname(__FILE__), "..", "app", "controllers")
$LOAD_PATH << File.join(File.dirname(__FILE__), "..", "app", "models")
module Blog
class Application < Simplemvc::Application
end
end
require "simplemvc/version"
module Simplemvc
# Your code goes here...
class Application
def call(env)
if env["PATH_INFO"] == "/"
return [ 302, {"Location" => "/pages/about"}, []]
end
if env["PATH_INFO"] == "/favicon.ico"
return [ 302, {}, []]
end
controller_class, action = get_controller_and_action(env)
response = controller_class.new.send(action)
[200, {"Content-Type" => "text/html"}, [response]]
end
def get_controller_and_action(env)
_, controller_name, action = env["PATH_INFO"].split("/")
controller_name = controller_name.capitalize + "Controller"
[Object.const_get(controller_name), action]
end
end
end
gem install erubis
Create the controller:
class MyPagesController < Simplemvc::Controller
attr_reader :name
def about
render :about, name: "Rem", last_name: "Zolotykh"
end
def tell_me
@name = "Rem"
end
end
run app
For more details visit Ruby MVC Framework From Scratch.
Download attached files