How to create a shared library in C on Linux
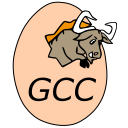
headers/foo.h:
#ifndef foo_h__
#define foo_h__
extern void foo(void);
#endif // foo_h__
utils/foo.c:
#include <stdio.h>
void foo(void)
{
puts("Hello, I am a shared library.");
}
main.c:
#include <stdio.h>
#include "headers/foo.h"
int main(void)
{
puts("This is a shared library test...");
foo();
return 0;
}
Step 1: Compiling with Position Independent Code
Inside the utils directory we need to compile our library source code into position-independent code (PIC):
gcc -c -Wall -Werror -fpic foo.c
Step 2: Creating a shared library from an object file
Now we need to actually turn this object file into a shared library. We will call it libfoo.so:
gcc -shared -o libfoo.so foo.o
Step 3: Making the library available at runtime
sudo mv libfoo.so /usr/lib/
Now the file is in a standard location, we need to tell the loader it is available for use, so let us update the cache:
sudo ldconfig
That should create a link to our shared library and update the cache so it is available for immediate use. Let us double check:
ldconfig -p | grep foo
Link our executable. Notice we do not need the -l option since our library is stored in a default location:
gcc -Wall -o test main.c -lfoo
Let us make sure we are using the /usr/lib instance of our library using ldd:
ldd test | grep foo
libfoo.so => /usr/lib/libfoo.so (0x00a42000)
Good, now let us run it:
./test
This is a shared library test...
Hello, I am a shared library.
That about wraps it up. We have covered how to build a shared library.