Type hinting in PHP
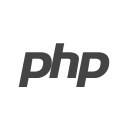
Type-hinting allows you to enforce specific types in your code. The code below tells the script to enforce strict types:
declare(strict_types=1);
Note: The strict_types declaration must be the very first statement in the script.
As of PHP 8.0, PHP has thirteen different types you can specify for declarations in your code. Let's take a look at each of them below:
- string - Value must be a string.
- int - Value must be an integer.
- float - Value must be a floating-point number.
- bool - Value must be Boolean (i.e., either true or false).
- array - Value must be an array.
- iterable - Value must be an array or object that can be used with the foreach loop.
- callable - Value must be a callable function.
- parent - Value must be an instance of the parent to the defining class. This can only be used on class and instance methods.
- self - Value must be either an instance of the class that defines the method or a child of the class. This can only be used on class and instance methods.
- interface name - Value must be an object that implements the given interface.
- class name - Value must be an instance of the given class name.
- mixed - Value can be any type.
- void - Value must be nothing. It can only be used in function returns.
Type-Hinting Function Parameters
function add(int $a, int $b) {
return $a + $b;
}
Type-Hinting Function Returns
function add(int $a, int $b): int {
return $a + $b;
}
Sometimes, you might not want to return anything from a function; if you would like to enforce this, you can use the void type:
function like(): void {
$post->likes + 1;
return;
}
Alternatively, you might want to return the instance of the object that defines a function from the same function. You can use the static type for this purpose:
class Person
{
public function returnPerson(): static
{
return new Person();
}
}
The code above defines a class, Person, with a function, returnPerson, that returns a Person object.
Nullable Types
function greeting(?string $username) : ?string
{
if ($username) {
return "Hello, $username!";
}
return null;
}
Union Types
function formatPrice(float | int $price): string
{
return '$' . number_format($price, 2);
}
Type-Hinting Class Properties
class Person
{
public string $name;
public int $age;
public float $height;
public bool $is_married;
public function __construct($name, $age, $height, $is_married)
{
$this->name = $name;
$this->age = $age;
$this->height = $height;
$this->is_married = $is_married;
}
}
The callable Type
function sortArray(callable $sort_function, array $array)
{
$sort_function($array);
return $array;
}
function bubbleSort(array $array): array
{
$sorted = false;
while (!$sorted) {
$sorted = true;
for ($i = 0; $i < count($array) - 1; $i++) {
if ($array[$i] > $array[$i + 1]) {
$temp = $array[$i];
$array[$i] = $array[$i + 1];
$array[$i + 1] = $temp;
$sorted = false;
}
}
}
print_r($array);
return $array;
}
sortArray('bubbleSort', [1, 3, 2, 5, 4]);
Constructor property promotion
class Customer
{
public function __construct(
public string $name,
public string $email,
public DateTimeImmutable $birth_date,
) {}
}