JPA annotations
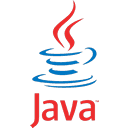
The Java Persistence API (JPA) is a Java application programming interface specification that describes the management of relational data in applications using Java Platform, Standard Edition and Java Platform, Enterprise Edition. This article provides you with 89 JPA mapping annotations for quick reference and/or summary. Let’s get started!
JPA annotations
1. @Access
The @Access
annotation is used to specify the access type of the associated entity class, mapped superclass, or embeddable class, or entity attribute.
See the Access type section for more info.
2. @AssociationOverride
The @AssociationOverride
annotation is used to override an association mapping (e.g. @ManyToOne
, @OneToOne
, @OneToMany
, @ManyToMany
) inherited from a mapped superclass or an embeddable.
See the Overriding Embeddable types section for more info.
3. @AssociationOverrides
The @AssociationOverrides
is used to group several @AssociationOverride
annotations.
4. @AttributeOverride
The @AttributeOverride
annotation is used to override an attribute mapping inherited from a mapped superclass or an embeddable.
See the Overriding Embeddable types section for more info.
5. @AttributeOverrides
The @AttributeOverrides
is used to group several @AttributeOverride
annotations.
6. @Basic
The @Basic
annotation is used to map a basic attribute type to a database column.
See the Basic types chapter for more info.
7. @Cacheable
The @Cacheable
annotation is used to specify whether an entity should be stored in the second-level cache.
If the persistence.xml
shared-cache-mode
XML attribute is set to ENABLE_SELECTIVE
, then only the entities annotated with the @Cacheable
are going to be stored in the second-level cache.
If shared-cache-mode
XML attribute value is DISABLE_SELECTIVE
, then the entities marked with the @Cacheable
annotation are not going to be stored in the second-level cache, while all the other entities are stored in the cache.
See the Caching chapter for more info.
8. @CollectionTable
The @CollectionTable
annotation is used to specify the database table that stores the values of a basic or an embeddable type collection.
See the Collections of embeddable types section for more info.
9. @Column
The @Column
annotation is used to specify the mapping between a basic entity attribute and the database table column.
See the @Column
annotation section for more info.
10. @ColumnResult
The @ColumnResult
annotation is used in conjunction with the @SqlResultSetMapping
or @ConstructorResult
annotations to map a SQL column for a given SELECT query.
See the Entity associations with named native queries section for more info.
11. @ConstructorResult
The @ConstructorResult
annotation is used in conjunction with the @SqlResultSetMapping
annotations to map columns of a given SELECT query to a certain object constructor.
See the Multiple scalar values NamedNativeQuery
with ConstructorResult
section for more info.
12. @Convert
The @Convert
annotation is used to specify the AttributeConverter
implementation used to convert the currently annotated basic attribute.
If the AttributeConverter
uses autoApply
, then all entity attributes with the same target type are going to be converted automatically.
See the AttributeConverter
section for more info.
13. @Converter
The @Converter
annotation is used to specify that the current annotate AttributeConverter
implementation can be used as a JPA basic attribute converter.
If the autoApply
attribute is set to true
, then the JPA provider will automatically convert all basic attributes with the same Java type as defined by the current converter.
See the AttributeConverter
section for more info.
14. @Converts
See the AttributeConverter
section for more info.
15. @DiscriminatorColumn
The @DiscriminatorColumn
annotation is used to specify the discriminator column name and the discriminator type for the SINGLE_TABLE
and JOINED
Inheritance strategies.
See the Discriminator section for more info.
16. @DiscriminatorValue
The @DiscriminatorValue
annotation is used to specify what value of the discriminator column is used for mapping the currently annotated entity.
See the Discriminator section for more info.
17. @ElementCollection
The @ElementCollection
annotation is used to specify a collection of a basic or embeddable types.
See the Collections section for more info.
18. @Embeddable
The @Embeddable
annotation is used to specify embeddable types. Like basic types, embeddable types do not have any identity, being managed by their owning entity.
See the Embeddables section for more info.
19. @Embedded
The @Embedded
annotation is used to specify that a given entity attribute represents an embeddable type.
See the Embeddables section for more info.
20. @EmbeddedId
The @EmbeddedId
annotation is used to specify the entity identifier is an embeddable type.
See the Composite identifiers with @EmbeddedId
section for more info.
21. @Entity
The @Entity
annotation is used to specify that the currently annotate class represents an entity type.
Unlike basic and embeddable types, entity types have an identity and their state is managed by the underlying Persistence Context.
See the Entity section for more info.
22. @EntityListeners
The @EntityListeners
annotation is used to specify an array of callback listener classes that are used by the currently annotated entity.
See the JPA callbacks section for more info.
23. @EntityResult
The @EntityResult
annotation is used with the @SqlResultSetMapping
annotation to map the selected columns to an entity.
See the Entity associations with named native queries section for more info.
24. @Enumerated
The @Enumerated
annotation is used to specify that an entity attribute represents an enumerated type.
See the @Enumerated
basic type section for more info.
25. @ExcludeDefaultListeners
The @ExcludeDefaultListeners
annotation is used to specify that the currently annotated entity skips the invocation of any default listener.
See the Exclude default entity listeners section for more info.
26. @ExcludeSuperclassListeners
The @ExcludeSuperclassListeners
annotation is used to specify that the currently annotated entity skips the invocation of listeners declared by its superclass.
See the Exclude default entity listeners section for more info.
27. @FieldResult
The @FieldResult
annotation is used with the @EntityResult
annotation to map the selected columns to the fields of some specific entity.
See the Entity associations with named native queries section for more info.
28. @ForeignKey
The @ForeignKey
annotation is used to specify the associated foreign key of a @JoinColumn
mapping.
The @ForeignKey
annotation is only used if the automated schema generation tool is enabled, in which case, it allows you to customize the underlying foreign key definition.
See the @ManyToOne
with @ForeignKey
section for more info.
29. @GeneratedValue
The @GeneratedValue
annotation specifies that the entity identifier value is automatically generated using an identity column, a database sequence, or a table generator.
Hibernate supports the @GeneratedValue
mapping even for UUID
identifiers.
See the Automatically-generated identifiers section for more info.
30. @Id
The @Id
annotation specifies the entity identifier.
An entity must always have an identifier attribute which is used when loading the entity in a given Persistence Context.
See the Identifiers section for more info.
31. @IdClass
The @IdClass
annotation is used if the current entity defined a composite identifier.
A separate class encapsulates all the identifier attributes, which are mirrored by the current entity mapping.
See the Composite identifiers with @IdClass
section for more info.
32. @Index
The @Index
annotation is used by the automated schema generation tool to create a database index.
See the Columns index chapter for more info.
33. @Inheritance
The @Inheritance
annotation is used to specify the inheritance strategy of a given entity class hierarchy.
See the Inheritance section for more info.
34. @JoinColumn
The @JoinColumn
annotation is used to specify the FOREIGN KEY column used when joining an entity association or an embeddable collection.
See the @ManyToOne
with @JoinColumn
section for more info.
35. @JoinColumns
The @JoinColumns
annotation is used to group multiple @JoinColumn
annotations, which are used when mapping entity association or an embeddable collection using a composite identifier
36. @JoinTable
The @JoinTable
annotation is used to specify the link table between two other database tables.
See the @JoinTable
mapping section for more info.
37. @Lob
The @Lob
annotation is used to specify that the currently annotated entity attribute represents a large object type.
See the BLOB
mapping section for more info.
38. @ManyToMany
The @ManyToMany
annotation is used to specify a many-to-many database relationship.
See the @ManyToMany
mapping section for more info.
39. @ManyToOne
The @ManyToOne
annotation is used to specify a many-to-one database relationship.
See the @ManyToOne
mapping section for more info.
40. @MapKey
The @MapKey
annotation is used to specify the key of a java.util.Map
association for which the key type is either the primary key or an attribute of the entity which represents the value of the map.
See the @MapKey
mapping section for more info.
41. @MapKeyClass
The @MapKeyClass
annotation is used to specify the type of the map key of a java.util.Map
associations.
See the @MapKeyClass
mapping section for more info.
42. @MapKeyColumn
The @MapKeyColumn
annotation is used to specify the database column which stores the key of a java.util.Map
association for which the map key is a basic type.
See the @MapKeyType
mapping section for an example of @MapKeyColumn
annotation usage.
43. @MapKeyEnumerated
The @MapKeyEnumerated
annotation is used to specify that the key of java.util.Map
association is a Java Enum.
See the @MapKeyEnumerated
mapping section for more info.
44. @MapKeyJoinColumn
The @MapKeyJoinColumn
annotation is used to specify that the key of java.util.Map
association is an entity association.
The map key column is a FOREIGN KEY in a link table that also joins the Map
owner’s table with the table where the Map
value resides.
See the @MapKeyJoinColumn
mapping section for more info.
45. @MapKeyJoinColumns
The @MapKeyJoinColumns
annotation is used to group several @MapKeyJoinColumn
mappings when the java.util.Map
association key uses a composite identifier.
46. @MapKeyTemporal
The @MapKeyTemporal
annotation is used to specify that the key of java.util.Map
association is a @TemporalType
(e.g. DATE
, TIME
, TIMESTAMP
).
See the @MapKeyTemporal
mapping section for more info.
47. @MappedSuperclass
The @MappedSuperclass
annotation is used to specify that the currently annotated type attributes are inherited by any subclass entity.
See the @MappedSuperclass
section for more info.
48. @MapsId
The @MapsId
annotation is used to specify that the entity identifier is mapped by the currently annotated @ManyToOne
or @OneToOne
association.
See the @MapsId
mapping section for more info.
49. @NamedAttributeNode
The @NamedAttributeNode
annotation is used to specify each individual attribute node that needs to be fetched by an Entity Graph.
See the Fetch graph section for more info.
50. @NamedEntityGraph
The @NamedEntityGraph
annotation is used to specify an Entity Graph that can be used by an entity query to override the default fetch plan.
See the Fetch graph section for more info.
51. @NamedEntityGraphs
The @NamedEntityGraphs
annotation is used to group multiple @NamedEntityGraph
annotations.
52. @NamedNativeQueries
The @NamedNativeQueries
annotation is used to group multiple @NamedNativeQuery
annotations.
See the Custom CRUD mapping section for more info.
53. @NamedNativeQuery
The @NamedNativeQuery
annotation is used to specify a native SQL query that can be retrieved later by its name.
See the Custom CRUD mapping section for more info.
54. @NamedQueries
The @NamedQueries
annotation is used to group multiple @NamedQuery
annotations.
55. @NamedQuery
The @NamedQuery
annotation is used to specify a JPQL query that can be retrieved later by its name.
See the @NamedQuery
section for more info.
56. @NamedStoredProcedureQueries
The @NamedStoredProcedureQueries
annotation is used to group multiple @NamedStoredProcedureQuery
annotations.
57. @NamedStoredProcedureQuery
The @NamedStoredProcedureQuery
annotation is used to specify a stored procedure query that can be retrieved later by its name.
See the Using named queries to call stored procedures section for more info.
58. @NamedSubgraph
The @NamedSubgraph
annotation used to specify a subgraph in an Entity Graph.
See the Fetch subgraph section for more info.
59. @OneToMany
The @OneToMany
annotation is used to specify a one-to-many database relationship.
See the @OneToMany
mapping section for more info.
60. @OneToOne
The @OneToOne
annotation is used to specify a one-to-one database relationship.
See the @OneToOne
mapping section for more info.
61. @OrderBy
The @OrderBy
annotation is used to specify the entity attributes used for sorting when fetching the currently annotated collection.
See the @OrderBy
mapping section for more info.
62. @OrderColumn
The @OrderColumn
annotation is used to specify that the current annotation collection order should be materialized in the database.
See the @OrderColumn
mapping section for more info.
63. @PersistenceContext
The @PersistenceContext
annotation is used to specify the EntityManager
that needs to be injected as a dependency.
See the @PersistenceContext
mapping section for more info.
64. @PersistenceContexts
The @PersistenceContexts
annotation is used to group multiple @PersistenceContext
annotations.
65. @PersistenceProperty
The @PersistenceProperty
annotation is used by the @PersistenceContext
annotation to declare JPA provider properties that are passed to the underlying container when the EntityManager
instance is created.
See the @PersistenceProperty
mapping section for more info.
66. @PersistenceUnit
The @PersistenceUnit
annotation is used to specify the EntityManagerFactory
that needs to be injected as a dependency.
See the @PersistenceUnit
mapping section for more info.
67. @PersistenceUnits
The @PersistenceUnits
annotation is used to group multiple @PersistenceUnit
annotations.
68. @PostLoad
The @PostLoad
annotation is used to specify a callback method that fires after an entity is loaded.
See the JPA callbacks section for more info.
69. @PostPersist
The @PostPersist
annotation is used to specify a callback method that fires after an entity is persisted.
See the JPA callbacks section for more info.
70. @PostRemove
The @PostRemove
annotation is used to specify a callback method that fires after an entity is removed.
See the JPA callbacks section for more info.
71. @PostUpdate
The @PostUpdate
annotation is used to specify a callback method that fires after an entity is updated.
See the JPA callbacks section for more info.
72. @PrePersist
The @PrePersist
annotation is used to specify a callback method that fires before an entity is persisted.
See the JPA callbacks section for more info.
73. @PreRemove
The @PreRemove
annotation is used to specify a callback method that fires before an entity is removed.
See the JPA callbacks section for more info.
74. @PreUpdate
The @PreUpdate
annotation is used to specify a callback method that fires before an entity is updated.
See the JPA callbacks section for more info.
75. @PrimaryKeyJoinColumn
The @PrimaryKeyJoinColumn
annotation is used to specify that the primary key column of the currently annotated entity is also a foreign key to some other entity
(e.g. a base class table in a JOINED
inheritance strategy, the primary table in a secondary table mapping, or the parent table in a @OneToOne
relationship).
See the @PrimaryKeyJoinColumn
mapping section for more info.
76. @PrimaryKeyJoinColumns
The @PrimaryKeyJoinColumns
annotation is used to group multiple @PrimaryKeyJoinColumn
annotations.
77. @QueryHint
The @QueryHint
annotation is used to specify a JPA provider hint used by a @NamedQuery
or a @NamedNativeQuery
annotation.
See the @QueryHint
section for more info.
78. @SecondaryTable
The @SecondaryTable
annotation is used to specify a secondary table for the currently annotated entity.
See the @SecondaryTable
mapping section for more info.
79. @SecondaryTables
The @SecondaryTables
annotation is used to group multiple @SecondaryTable
annotations.
80. @SequenceGenerator
The @SequenceGenerator
annotation is used to specify the database sequence used by the identifier generator of the currently annotated entity.
See the @SequenceGenerator
mapping section for more info.
81. @SqlResultSetMapping
The @SqlResultSetMapping
annotation is used to specify the ResultSet
mapping of a native SQL query or stored procedure.
See the SqlResultSetMapping
mapping section for more info.
82. @SqlResultSetMappings
The @SqlResultSetMappings
annotation is group multiple @SqlResultSetMapping
annotations.
83. @StoredProcedureParameter
The @StoredProcedureParameter
annotation is used to specify a parameter of a @NamedStoredProcedureQuery
.
See the Using named queries to call stored procedures section for more info.
84. @Table
The @Table
annotation is used to specify the primary table of the currently annotated entity.
See the @Table
mapping section for more info.
85. @TableGenerator
The @TableGenerator
annotation is used to specify the database table used by the identity generator of the currently annotated entity.
See the @TableGenerator
mapping section for more info.
86. @Temporal
The @Temporal
annotation is used to specify the TemporalType
of the currently annotated java.util.Date
or java.util.Calendar
entity attribute.
See the Basic temporal types chapter for more info.
87. @Transient
The @Transient
annotation is used to specify that a given entity attribute should not be persisted.
See the @Transient
mapping section for more info.
88. @UniqueConstraint
The @UniqueConstraint
annotation is used to specify a unique constraint to be included by the automated schema generator for the primary or secondary table associated with the currently annotated entity.
See the Columns unique constraint chapter for more info.
89. @Version
The @Version
annotation is used to specify the version attribute used for optimistic locking.
See the Optimistic locking mapping section for more info.