Apache Maven Fundamentals
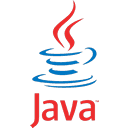
Apache Maven is a software project management and comprehension tool. Based on the concept of a project object model (POM), Maven can manage a project’s build, reporting and documentation from a central piece of information. Maven aims to gather current principles for best practices development.
Maven’s Objectives
Maven’s primary goal is to allow a developer to comprehend the complete state of a development effort in the shortest period of time. In order to attain this goal there are several areas of concern that Maven attempts to deal with:
- Making the build process easy
- Providing a uniform build system
- Providing quality project information
- Providing guidelines for best practices development
- Allowing transparent migration to new features
Maven Demo:
package com.maven;
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello World");
}
}
<project>
<modelVersion>4.0.0</modelVersion>
<artifactId>maven-hello-world</artifactId>
<groupId>com.maven</groupId>
<version>1.0</version>
<packaging>jar</packaging>
</project>
mvn compile
mvn package
Maven Standard Directory Layout
src/main/java | Application/Library sources |
src/main/resources | Application/Library resources |
src/main/resources-filtered | Application/Library resources which are filtered. (Starting with Maven 3.4.0, not yet released.) |
src/main/filters | Resource filter files |
src/main/webapp | Web application sources |
src/test/java | Test sources |
src/test/resources | Test resources |
src/test/resources-filtered | Test resources which are filtered by default. (Starting with Maven 3.4.0, not yet released.) |
src/test/filters | Test resource filter files |
src/it | Integration Tests (primarily for plugins) |
src/assembly | Assembly descriptors |
src/site | Site |
LICENSE.txt | Project's license |
NOTICE.txt | Notices and attributions required by libraries that the project depends on |
README.txt | Project's readme |
Inheritance
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd ">
<modelVersion>4.0.0</modelVersion>
<artifactId>organization-parent-pom</artifactId>
<groupId>com.maven</groupId>
<version>1.0.0</version>
<packaging>pom</packaging>
<name>Learning Maven Examples</name>
<description>This is a project used to demonstrate maven principles</description>
<url>http://course.maven.com</url>
<licenses>
<license>
<name>Apache License</name>
<comments>We are pretty good about sharing</comments>
</license>
</licenses>
</project>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd ">
<modelVersion>4.0.0</modelVersion>
<artifactId>maven-examples</artifactId>
<parent>
<artifactId>organization-parent-pom</artifactId>
<groupId>com.maven</groupId>
<version>1.0.0</version>
</parent>
</project>
Profiles
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd ">
<modelVersion>4.0.0</modelVersion>
<artifactId>maven-examples</artifactId>
<groupId>com.maven</groupId>
<version>1.0.0</version>
<build>
<directory>C:\Users\Andrei Pall\Desktop\Artifact Destination\development\</directory>
</build>
<profiles>
<profile>
<id>production</id>
<activation>
<property>
<name>env.PACKAGE_ENV</name>
<value>PROD</value>
</property>
</activation>
<build>
<directory>C:\Users\Andrei Pall\Desktop\Artifact Destination\production\</directory>
</build>
</profile>
</profiles>
</project>
mvn clean
mvn -Pproduction package
Generating Projects
mvn archetype:generate
Dependency Management
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.maven</groupId>
<artifactId>more-maven-examples</artifactId>
<version>1.0</version>
<packaging>jar</packaging>
<name>more-maven-examples</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Plugins Jar Plugin
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.maven</groupId>
<artifactId>more-maven-examples</artifactId>
<version>1.0</version>
<packaging>jar</packaging>
<name>more-maven-examples</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<build>
<pluginManagement>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.2</version>
<configuration>
<verbose>true</verbose>
</configuration>
</plugin>
<plugin>
<artifactId>maven-jar-plugin</artifactId>
<version>2.4</version>
<configuration>
<finalName>pomTest</finalName>
<forceCreation>true</forceCreation>
<excludes>
<exclude>**/ExcludeMe.class</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</pluginManagement>
</build>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Javadoc Plugin
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.maven</groupId>
<artifactId>more-maven-examples</artifactId>
<version>1.0</version>
<packaging>jar</packaging>
<name>more-maven-examples</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<build>
<plugins>
<plugin>
<artifactId>maven-javadoc-plugin</artifactId>
<groupId>org.apache.maven.plugins</groupId>
<version>2.10.1</version>
</plugin>
</plugins>
<pluginManagement>
<plugins>
<plugin>
<artifactId>maven-javadoc-plugin</artifactId>
<configuration>
<footer>This is the new footer</footer>
</configuration>
<executions>
<execution>
<phase>compile</phase>
<goals><goal>jar</goal></goals>
</execution>
</executions>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.2</version>
<configuration>
<verbose>true</verbose>
</configuration>
</plugin>
<plugin>
<artifactId>maven-jar-plugin</artifactId>
<version>2.4</version>
<configuration>
<finalName>pomTest</finalName>
<forceCreation>true</forceCreation>
<excludes>
<exclude>**/ExcludeMe.class</exclude>
</excludes>
</configuration>
</plugin>
<plugin>
<artifactId>maven-javadoc-plugin</artifactId>
<executions>
<execution>
<id>attach-javadocs</id>
<phase>compile</phase>
<goals>
<goal>jar</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</pluginManagement>
</build>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
War Plugin
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.maven</groupId>
<artifactId>web-maven-example</artifactId>
<packaging>war</packaging>
<version>1.0-SNAPSHOT</version>
<name>web-maven-example Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>2.6</version>
<configuration>
<warName>mavenWeb</warName>
</configuration>
</plugin>
</plugins>
</build>
</project>